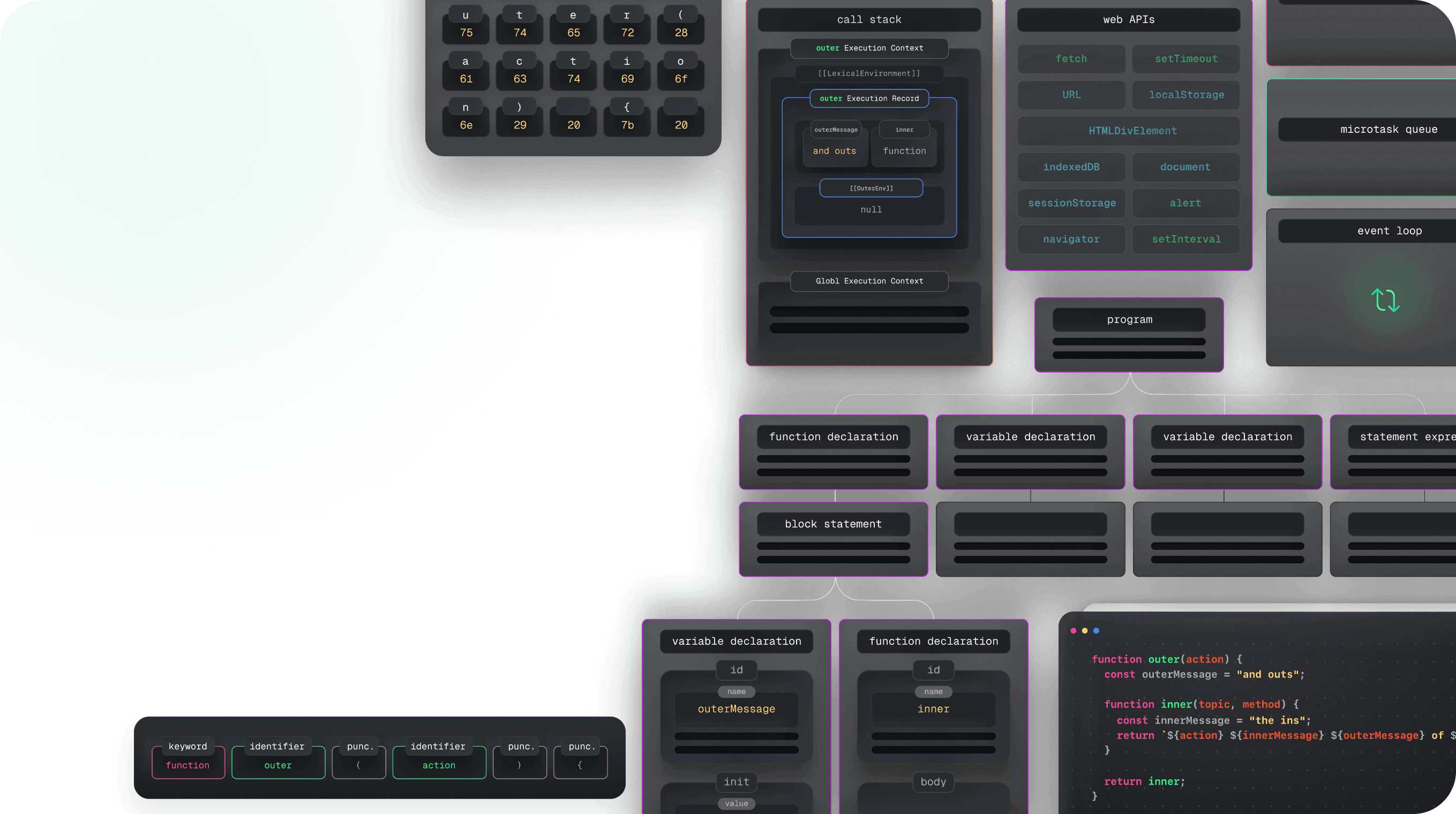

Deep Comprehensive Understanding of JavaScript Visualized

You probably won't have any trouble reading this code snippet.

What will be printed to the console when you run this code in the browser console?
The first thing printed will be Start
, then End
, then finally
Callback
. Easy, right?
So you know what will happen, but do you know why?
Most resources would give you a high-level explanation, perhaps involving a metaphor about a restaurant kitchen or a rollercoaster. But that doesn't really help you understand the nitty-gritty details of the Event Loop, the Web API, and the task queue, does it?
This illustrates one of the biggest pain points in the JavaScript ecosystem: the lack of resources that strike the perfect balance between depth and clarity.
On one end of the spectrum, you have tutorials and articles that barely skim the surface, leaving you with more questions than answers. On the other end, are explanations that are so dry and boring that they suck the joy out of learning.
Because of this, many developers barely scratch the surface of a language, relying more on pattern recognition and memorization rather than building a deep understanding of what's going on behind the scenes.
While you can certainly build a successful career without understanding the inner workings of JavaScript, you'll hit a ceiling at some point.
You'll struggle with debugging, optimization, and grasping advanced concepts. You'll find it hard to keep up with why trends and tools change, and you'll be limited in your ability to contribute to your work and the community at large.
Why settle for good, when you can be great?
You could be the developer that others turn to for help, the one who can explain complex concepts in a way that makes sense to everyone without relying on oversimplified metaphors.
You could understand the root cause behind bugs and performance issues, write more efficient code, and contribute to open-source projects with confidence. You could build your own libraries and frameworks, and even create your own tools to analyze and optimize your code.
That's where JavaScript Visualized comes in.
Introducing
JavaScript Visualized
JavaScript Visualized is designed to provide you with a deep, comprehensive understanding of JavaScript's internals, without relying on oversimplified metaphors or getting bogged down by boredom.
With JavaScript Visualized, you will:
- Gain the expertise to develop your own libraries and frameworks, understanding the foundational principles behind libraries and tools like React and Node.js
- Establish yourself as an indispensable JavaScript expert, armed with the knowledge to resolve complex issues and debug challenging code
- Write faster, more efficient code while minimizing errors, by leveraging JavaScript's built-in features for peak performance
- Experience indescribable satisfaction as concepts click into place, reminding you why you fell in love with code in the first place


Hi, I'm Lydia Hallie
I'm a software engineering consultant, educator, and creator of JavaScript Visualized. I love the challenge of creating in-depth technical explanations that go to the lowest level possible while still being enjoyable and easy to understand. JavaScript Visualized is the resource I wish I had when I was learning JavaScript's internals, and I'm excited to share it with you!
Here's a closer look at what you'll learn:
Parsing and Lexing
Dive deep into the world of code interpretation and uncover how JavaScript breaks down and understands your scripts. By learning about tokens and syntax errors, you'll lay a strong foundation for writing cleaner, error-free code.
Abstract Syntax Trees (ASTs)
Explore the hierarchical structure that represents your code's relationships and components. ASTs can even be used to create your own code analysis tools.
JavaScript Engine and Runtime
Peek behind the curtain at how your code is compiled, optimized, and executed in the browser and V8 engines.
Execution Context and Environments
Demystify variable scope, hoisting, and the execution stack by learning how JavaScript manages context and environments. Predict and control your code's behavior with confidence.
Closures and Scopes
Learn how closures reference the lexical environments in your code, and understand that hoisting isn't just moving variables to the top of your file.
Memory Management
Take control of memory usage through garbage collection and allocation strategies. Prevent leaks and optimize resource consumption for peak application performance.
Event Loop
Unravel the mysteries the event loop, call stack, and the task & microtask queues. Everything in JavaScript revolves around the event loop.
Promises
Gain a deeper understanding of what really goes on with asynchronous code in JavaScript. Learn how to create, consume, and chain promises to manage complex workflows, and what makes async/await tick.
Prototypes
Grasp the relationship between classes and prototypes, including how inheritance works in JavaScript.
Generators
Control function execution, manage data streams, and simplify complex workflows with the power of generators. Learn how to pause and resume functions at will.
Modules
Compare and contrast CommonJS, AMD, UMD, and ESM modules and their impact on your codebase. Organize your code while supporting async imports, tree shaking, and static analysis.
JavaScript Visualized is not a course that holds your hand through building an app or using a library. Increase your confidence by equipping yourself with the knowledge of how JavaScript's behind-the-scenes processes impact the code you write every day.
Deeper Understanding Awaits
Sign up today and unlock your full potential as a developer. Get ready to rekindle your passion for coding, advance your career, and make a lasting impact on the JavaScript community.
I respect your privacy. Unsubscribe at any time.
So, what about
the code snippet?
If you're still wondering why Start
, End
, and Callback
are printed in that
order even though logCallback()
is called first, the answer lies in how
setTimeout
interacts with the task queue and the event loop. In short, when
the callback function is pushed to the Web API, the setTimeout
function
without the callback is popped off the call stack. This allows logStart()
and
logEnd()
to be executed. Once the call stack is empty, the callback function
is pushed to the task queue, where it waits for the event loop to pick it up and
execute it before popping it off the call stack.